I have include the execution plan pictures for some of the queries so that you can see the difference
Don’t use * but list the columns
SELECT pub_name,city FROM dbo.publishers instead of SELECT * FROM dbo.publishers
If you would have a covering index on the columns pub_name and city then the table would not be accessed at all and all the data would be returned from the index. This would also reduce the logical reads. You can use STATISTICS IO to find out how many logical reads you would have
SELECT pub_name,city FROM dbo.publishers
Table 'Products'. Scan count 1, logical reads 1, physical reads 0, read-ahead reads 0.
SELECT * FROM dbo.publishers
Table 'Products'. Scan count 1, logical reads 7, physical reads 0, read-ahead reads 0.
Don’t use arithmetic operators on a column in the where clause
You will get an index scan instead of a seek
SELECT * FROM Orders WHERE OrderID*3 = 33000
SELECT * FROM Orders WHERE OrderID = 33000/3
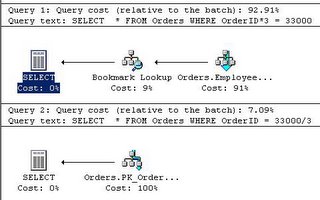
Don’t use functions on a column in the where clause
You will get an index scan instead of a seek
SELECT * FROM Orders WHERE LEFT(CustomerID,1) ='V'
SELECT * FROM Orders WHERE CustomerID LIKE 'V%'
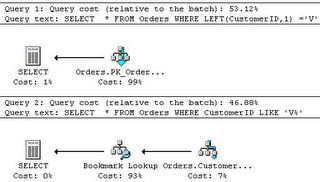
Don’t convert a datetime column in the where clause
SELECT * FROM Orders WHERE OrderDate = '1996-07-04'
SELECT * FROM Orders WHERE CONVERT(CHAR(10),OrderDate,120) = '1996-07-04'
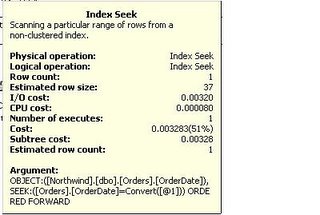
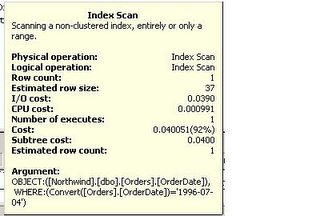
As you can see the query is many time faster if you don't convert
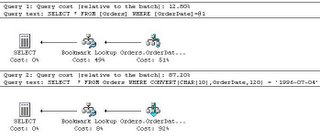
3 comments:
I'm relatively new to SQL. Why don't SQL compilers perform these optimisationsL? They seem like transformations easy to implement and detect the conditions under which they can apply.
Denis, you are absolutely right. These are optimizations specific to some relatively lame SQL query engine.
That said, some of these things are just really strange to do.
I also posted one blog entry related to common query performance problems....
http://mohitnayyar.blogspot.com/2007/08/microsoft-sql-server-common-query.html
- Mohit Nayyar
Post a Comment