Sometimes you want to have your money fields properly formatted with commas like this: 13,243,543.57
You can use the CONVERT function and give a value between 0 and 2 to the style and the format will be displayed based on that
Below is an example
DECLARE @v MONEY
SELECT @v = 1322323.6666
SELECT CONVERT(VARCHAR,@v,0) --1322323.67 Rounded but not formatted
SELECT CONVERT(VARCHAR,@v,1) --1,322,323.67 Formatted with commas
SELECT CONVERT(VARCHAR,@v,2) --1322323.6666 No formatting
If you have a decimal field it doesn't work with the convert function. The work around is to cast it to money
DECLARE @v2 DECIMAL (36,10)
SELECT @v2 = 13243543.56565656
SELECT CONVERT(VARCHAR,CONVERT(MONEY,@v2),1) --13,243,543.57 Formatted with commas
A blog about SQL Server, Books, Movies and life in general
Thursday, December 29, 2005
Friday, December 23, 2005
How To Resolve A Deadlock
I stumbled upon this SQL Server technical bulletin and it gives one example of how to resolve a deadlock. Typical methods you can use to resolve deadlocks include:
Adding and dropping indexes.
Adding index hints.
Modifying the application to access resources in a similar pattern.
Removing activity from the transaction like triggers. By default, triggers are transactional.
Keeping transactions as short as possible.
I won't go into details here since you can read it all at the following link (SQL Server technical bulletin - How to resolve a deadlock)
Also check out the following deadlock links
Deadlocking
Handling Deadlocks
Minimizing Deadlocks
Troubleshooting Deadlocks
Lock Compatibility
And of course if you really want to know everything about locking I recommend the following book (in PDF format): Hands-On SQL Server 2000 : Troubleshooting Locking and Blocking by Kalen Delaney (Inside SQL Server 2000)
Adding and dropping indexes.
Adding index hints.
Modifying the application to access resources in a similar pattern.
Removing activity from the transaction like triggers. By default, triggers are transactional.
Keeping transactions as short as possible.
I won't go into details here since you can read it all at the following link (SQL Server technical bulletin - How to resolve a deadlock)
Also check out the following deadlock links
Deadlocking
Handling Deadlocks
Minimizing Deadlocks
Troubleshooting Deadlocks
Lock Compatibility
And of course if you really want to know everything about locking I recommend the following book (in PDF format): Hands-On SQL Server 2000 : Troubleshooting Locking and Blocking by Kalen Delaney (Inside SQL Server 2000)
Wednesday, December 21, 2005
SQL Server 2005 System Table Map PDF Download
A couple of days back I reported that the current issue of SQL Server Magazine includes a poster of all the SQL Server 2005 System Tables. Well Microsoft has made this poster available as a PDF download now. You can get it here (SQL Server 2005 System Table Map)
Monday, December 19, 2005
SQL Query Optimizations
Below are a couple of small SQL query optimization tips
I have include the execution plan pictures for some of the queries so that you can see the difference
Don’t use * but list the columns
SELECT pub_name,city FROM dbo.publishers instead of SELECT * FROM dbo.publishers
If you would have a covering index on the columns pub_name and city then the table would not be accessed at all and all the data would be returned from the index. This would also reduce the logical reads. You can use STATISTICS IO to find out how many logical reads you would have
SELECT pub_name,city FROM dbo.publishers
Table 'Products'. Scan count 1, logical reads 1, physical reads 0, read-ahead reads 0.
SELECT * FROM dbo.publishers
Table 'Products'. Scan count 1, logical reads 7, physical reads 0, read-ahead reads 0.
Don’t use arithmetic operators on a column in the where clause
You will get an index scan instead of a seek
SELECT * FROM Orders WHERE OrderID*3 = 33000
SELECT * FROM Orders WHERE OrderID = 33000/3
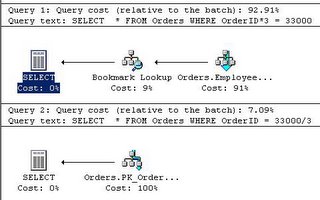
Don’t use functions on a column in the where clause
You will get an index scan instead of a seek
SELECT * FROM Orders WHERE LEFT(CustomerID,1) ='V'
SELECT * FROM Orders WHERE CustomerID LIKE 'V%'
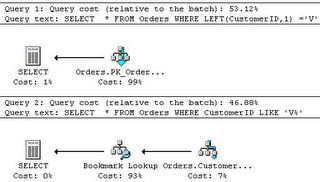
Don’t convert a datetime column in the where clause
SELECT * FROM Orders WHERE OrderDate = '1996-07-04'
SELECT * FROM Orders WHERE CONVERT(CHAR(10),OrderDate,120) = '1996-07-04'
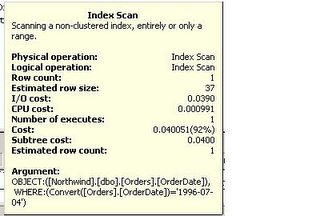
As you can see the query is many time faster if you don't convert
I have include the execution plan pictures for some of the queries so that you can see the difference
Don’t use * but list the columns
SELECT pub_name,city FROM dbo.publishers instead of SELECT * FROM dbo.publishers
If you would have a covering index on the columns pub_name and city then the table would not be accessed at all and all the data would be returned from the index. This would also reduce the logical reads. You can use STATISTICS IO to find out how many logical reads you would have
SELECT pub_name,city FROM dbo.publishers
Table 'Products'. Scan count 1, logical reads 1, physical reads 0, read-ahead reads 0.
SELECT * FROM dbo.publishers
Table 'Products'. Scan count 1, logical reads 7, physical reads 0, read-ahead reads 0.
Don’t use arithmetic operators on a column in the where clause
You will get an index scan instead of a seek
SELECT * FROM Orders WHERE OrderID*3 = 33000
SELECT * FROM Orders WHERE OrderID = 33000/3
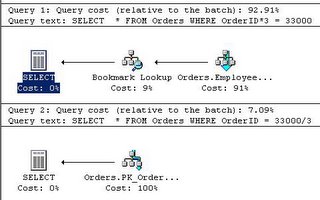
Don’t use functions on a column in the where clause
You will get an index scan instead of a seek
SELECT * FROM Orders WHERE LEFT(CustomerID,1) ='V'
SELECT * FROM Orders WHERE CustomerID LIKE 'V%'
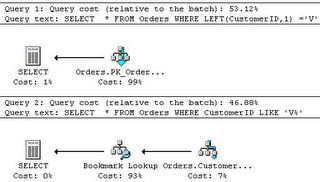
Don’t convert a datetime column in the where clause
SELECT * FROM Orders WHERE OrderDate = '1996-07-04'
SELECT * FROM Orders WHERE CONVERT(CHAR(10),OrderDate,120) = '1996-07-04'
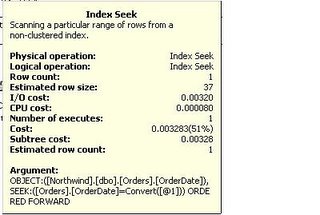
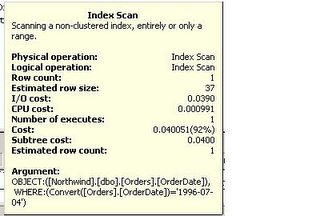
As you can see the query is many time faster if you don't convert
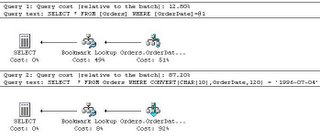
Thursday, December 15, 2005
Test SQL Server Login Permissions With SETUSER
Sometimes you get a request to create a login and you want to test the permissions before letting the user know that he can use the login. you just don't feel like login in and trying to run some SQL statements for every user.
With the SETUSER statement you can eliminate that. Here is some code that explains how to use it
--Create the user
EXEC sp_addlogin 'Albert', 'food', 'pubs'
EXEC sp_adduser 'Albert'
CREATE TABLE dbo.test(id INT identity,datefield DATETIME)
INSERT INTO test
SELECT GETDATE()
SELECT * FROM test -- as dbo
-- let's run the select as Albert
SETUSER 'Albert'
SELECT * FROM test -- as albert
Now you should get this error
Server: Msg 229, Level 14, State 5, Line 1
SELECT permission denied on object 'test', database 'pubs', owner 'dbo'.
execute just the SETUSER statement and you can drop Albert since the user will be reset to the original user
SETUSER
EXEC sp_dropuser 'Albert'
EXEC sp_droplogin 'Albert'
or close the query window and in another window execute the code below to drop Albert
You have to do this in another window since as Albert you don't have permissions to do this
EXEC sp_dropuser 'Albert'
EXEC sp_droplogin 'Albert'
With the SETUSER statement you can eliminate that. Here is some code that explains how to use it
--Create the user
EXEC sp_addlogin 'Albert', 'food', 'pubs'
EXEC sp_adduser 'Albert'
CREATE TABLE dbo.test(id INT identity,datefield DATETIME)
INSERT INTO test
SELECT GETDATE()
SELECT * FROM test -- as dbo
-- let's run the select as Albert
SETUSER 'Albert'
SELECT * FROM test -- as albert
Now you should get this error
Server: Msg 229, Level 14, State 5, Line 1
SELECT permission denied on object 'test', database 'pubs', owner 'dbo'.
execute just the SETUSER statement and you can drop Albert since the user will be reset to the original user
SETUSER
EXEC sp_dropuser 'Albert'
EXEC sp_droplogin 'Albert'
or close the query window and in another window execute the code below to drop Albert
You have to do this in another window since as Albert you don't have permissions to do this
EXEC sp_dropuser 'Albert'
EXEC sp_droplogin 'Albert'
Wednesday, December 14, 2005
SQL Server 2005 T-SQL Recipes: A Problem-Solution Approach
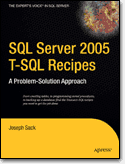
This book has been published it is 768 pages and published by Apress and you can order it from amazon
You can download chapter 6 here to get a feel for the book
Need to brush up on specific SQL Server tasks, procedures, or Transact-SQL commands? Not finding what you need from SQL Server books online? Or perhaps you just want to familiarize yourself with the practical application of new T-SQL–related features. SQL Server 2005 T-SQL Recipes: A Problem-Solution Approach is an ideal book, whatever your level as a DBA or developer.
This “no-fluff” desk reference offers direct access to the information you need to get the job done. It covers basic T-SQL data manipulation, the use of stored procedures, triggers and UDFs, and advanced T-SQL techniques for database security and maintenance. It also provides hundreds of practical recipes that describe the utilities of features and functions, with a minimim of background theory.
Additionally, this book provides “how-to” answers to common SQL Server T-SQL questions, conceptual overviews, and highlights of new features introduced in SQL Server 2005. It also features concise T-SQL syntax examples, and you can use the book to prepare for a SQL Server-related job interview or certification test.
Below is the table of contents
CHAPTER 1 SELECT
CHAPTER 2 INSERT, UPDATE, DELETE
CHAPTER 3 Transactions, Locking, Blocking, and Deadlocking
CHAPTER 4 Tables
CHAPTER 5 Indexes
CHAPTER 6 Full-Text Search
CHAPTER 7 Views
CHAPTER 8 SQL Server Functions
CHAPTER 9 Conditional Processing, Control-Of-Flow, and Cursors
CHAPTER 10 Stored Procedures
CHAPTER 11 User-Defined Functions and Types
CHAPTER 12 Triggers
CHAPTER 13 CLR Integration
CHAPTER 14 XML
CHAPTER 15 Web Services
CHAPTER 16 Error Handling
CHAPTER 17 Principals
CHAPTER 18 Securables and Permissions
CHAPTER 19 Encryption
CHAPTER 20 Service Broker
CHAPTER 21 Configuring and Viewing SQL Server Options
CHAPTER 22 Creating and Configuring Databases
CHAPTER 23 Database Integrity and Optimization
CHAPTER 24 Maintaining Database Objects and Object Dependencies
CHAPTER 25 Database Mirroring
CHAPTER 26 Database Snapshots
CHAPTER 27 Linked Servers and Distributed Queries
CHAPTER 28 Performance Tuning
CHAPTER 29 Backup and Recovery
Tuesday, December 13, 2005
SQL Server 2005 Free E-Learning
Whether you are interested in database administration, database development, or business intelligence, there is some free * E-Learning to help you get up to speed on the newest features of the software. The E-Learning courses, valued at $99 each, are an effective way to learn on your own schedule and feature hands-on virtual labs that provide an in-depth, online training experience.
Database Administrator
2936: Installing and Securing Microsoft SQL Server 2005
2937: Administering and Monitoring Microsoft SQL Server 2005
2938: Data Availability Features in Microsoft SQL Server 2005
Database Developer
2939: Programming Microsoft SQL Server 2005
2940: Building Services and Notifications Using Microsoft SQL Server 2005
2941: Creating the Data Access Tier Using Microsoft SQL Server 2005
Business Intelligence Developer
2942: New Features of Microsoft SQL Server 2005 Analysis Services
2943: Updating Your Data ETL Skills to Microsoft SQL Server 2005 Integration Services
2944: Updating Your Reporting Skills to Microsoft SQL Server 2005 Reporting Services
* Microsoft E-Learning for SQL Server 2005 is free until November 1, 2006. Note that this is a limited time offer and Internet connection time charges may apply.
Database Administrator
2936: Installing and Securing Microsoft SQL Server 2005
2937: Administering and Monitoring Microsoft SQL Server 2005
2938: Data Availability Features in Microsoft SQL Server 2005
Database Developer
2939: Programming Microsoft SQL Server 2005
2940: Building Services and Notifications Using Microsoft SQL Server 2005
2941: Creating the Data Access Tier Using Microsoft SQL Server 2005
Business Intelligence Developer
2942: New Features of Microsoft SQL Server 2005 Analysis Services
2943: Updating Your Data ETL Skills to Microsoft SQL Server 2005 Integration Services
2944: Updating Your Reporting Skills to Microsoft SQL Server 2005 Reporting Services
* Microsoft E-Learning for SQL Server 2005 is free until November 1, 2006. Note that this is a limited time offer and Internet connection time charges may apply.
Monday, December 12, 2005
Fun With SQL Server Update Triggers
Below is some code that will show how to test for updated field values in an update trigger. As you can see the IF UPDATE (field) is true even when the values don’t change. Another thing to keep in mind is that if a value changes from NULL to something else and vice-versa, and you are comparing deleted and inserted tables without using COALESCE or ISNULL it won’t return those rows. Run the code below to see what I mean
CREATE TABLE TestTrigger (TestID INT identity,
name VARCHAR(20),
value DECIMAL(12,2) ,
CONSTRAINT chkPositiveValue CHECK (value > 0.00) )
INSERT INTO TestTrigger
SELECT 'SQL',500.23
CREATE TRIGGER trTest
ON TestTrigger
FOR UPDATE
AS
IF @@ROWCOUNT =0
RETURN
IF UPDATE(value)
BEGIN
SELECT '1', * FROM deleted d JOIN inserted i ON d.testid =i.testid
SELECT '2',* FROM deleted d JOIN inserted i ON d.testid =i.testid
AND i.value <> d.value
SELECT '3',* FROM deleted d JOIN inserted i ON d.testid =i.testid
AND COALESCE(i.value,-1) <> COALESCE(d.value,-1)
END
GO
--Let's update the value to 100
UPDATE TestTrigger SET value = 100 WHERE testid =1
--we get back all 3 rows
--Let's run the same statement
UPDATE TestTrigger SET value = 100 WHERE testid =1
--we get back the first row
--Let's really update
UPDATE TestTrigger SET value = 200 WHERE testid =1
--we get back all 3 rows
--Let's update with NULL
UPDATE TestTrigger SET value =NULL WHERE testid =1
--we get back rows 1 and 3, row 2 is not returned because it can't compare it
--Let's update with NULL again
UPDATE TestTrigger SET value =NULL WHERE testid =1
--we get back row 1
--Let's update with 300
UPDATE TestTrigger SET value =300 WHERE testid =1
--we get back rows 1 and 3, row 2 doesn't return because it can't compare NULL to 300
--Let's update with 500
UPDATE TestTrigger SET value =500 WHERE testid =1
--we get back all 3 rows
CREATE TABLE TestTrigger (TestID INT identity,
name VARCHAR(20),
value DECIMAL(12,2) ,
CONSTRAINT chkPositiveValue CHECK (value > 0.00) )
INSERT INTO TestTrigger
SELECT 'SQL',500.23
CREATE TRIGGER trTest
ON TestTrigger
FOR UPDATE
AS
IF @@ROWCOUNT =0
RETURN
IF UPDATE(value)
BEGIN
SELECT '1', * FROM deleted d JOIN inserted i ON d.testid =i.testid
SELECT '2',* FROM deleted d JOIN inserted i ON d.testid =i.testid
AND i.value <> d.value
SELECT '3',* FROM deleted d JOIN inserted i ON d.testid =i.testid
AND COALESCE(i.value,-1) <> COALESCE(d.value,-1)
END
GO
--Let's update the value to 100
UPDATE TestTrigger SET value = 100 WHERE testid =1
--we get back all 3 rows
--Let's run the same statement
UPDATE TestTrigger SET value = 100 WHERE testid =1
--we get back the first row
--Let's really update
UPDATE TestTrigger SET value = 200 WHERE testid =1
--we get back all 3 rows
--Let's update with NULL
UPDATE TestTrigger SET value =NULL WHERE testid =1
--we get back rows 1 and 3, row 2 is not returned because it can't compare it
--Let's update with NULL again
UPDATE TestTrigger SET value =NULL WHERE testid =1
--we get back row 1
--Let's update with 300
UPDATE TestTrigger SET value =300 WHERE testid =1
--we get back rows 1 and 3, row 2 doesn't return because it can't compare NULL to 300
--Let's update with 500
UPDATE TestTrigger SET value =500 WHERE testid =1
--we get back all 3 rows
Labels:
Howto,
SQL Server 2000,
SQL Server 2005,
SQL Server 2008,
tip,
trick,
Triggers
Thursday, December 08, 2005
Keep Your Statistics Up To Date
I had a scheduled job that runs every hour and took about a minute to complete. Somehow for no good reason this job suddenly takes hours and hours to finish. There was nothing done to the server, no service packs or patches. I checked fragmentation with DBCC SHOWCONTIG and it looked fine. I checked for blocking by running sp_who2 and there was no blocking going on. Now I was puzzled, what could have caused this? The table is not huge, about 50000 rows. Then I decided to update the statistics by running UPDATE STATISTICS and the problem went away immediately. The strange part is that there was such a huge difference in excution time, if it was double or triple I would understand but this was hunderds times slower. I don't have auto update statistics enabled because I have huge tables and can not have SQL server running sp_updatestats in the middle of the day. I have added this one table to the statistics job that I currently have and will monitor if the stats are up to date
SQL Server 2005 System View Poster

Objects, Types and Indexes
Trace and Eventing
Linked Servers
Common Language Runtime
Partitioning
Databases and Storage
Execution Environment
Service Broker
Security
Transaction Information
Serveriwide Configurations
Server-wide Information
SQL Server 2005 December BOL Update And Samples
Updated versions of Books On Line and Sample Databases are available from the links below
SQL Server 2005 Books Online (December 2005)
SQL Server 2005 Samples and Sample Databases (December 2005).
SQL Server 2005 Books Online (December 2005)
SQL Server 2005 Samples and Sample Databases (December 2005).
Monday, December 05, 2005
SQL Server Site Geo Map For November
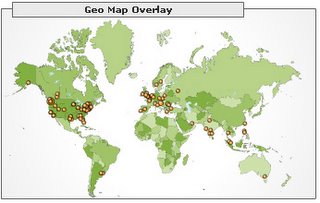
These are the countries where the bulk of the visitors for this blog are coming from. As you can see most off the visitors are from the US and Europe, Asia is in third place. When I look at the maps day by day I also see some visitors from Africa and the Middle East but you need more than one visitor per city in order for it to show up in the monthly map. I only started Google Analytics at the end of November so it will be interesting to see if December will be much different
SQL Server 2005 Master Database System Views
Below is a list of the SQL Server 2000 system tables and the corresponding SQL Server 2005 system views in the master database
SQL Server 2000 System Table SQL Server 2005 System View
sysaltfiles....................sys.master_files
syscacheobjects................sys.dm_exec_cached_plans
syscharsets....................sys.charsets
sysconfigures..................sys.configurations
syscurconfigs..................sys.configurations
sysdatabases...................sys.databases
sysdevices.....................sys.backup_devices
syslanguages...................sys.languages
syslockinfo....................sys.dm_tran_locks
syslocks.......................sys.dm_tran_locks
syslogins......................sys.server_principals
sysmessages....................sys.messages
sysoledbusers..................sys.linked_logins
sysopentapes...................sys.dm_io_backup_tapes
sysperfinfo....................sys.dm_os_performance_counters
sysprocesses...................sys.dm_exec_connections
...............................sys.dm_exec_sessions
...............................sys.dm_exec_requests
sysremotelogins................sys.remote_logins
sysservers.....................sys.servers
Saturday, December 03, 2005
November SQL Google Searches
These are the top SQL Searches on this site for the month of November. I have left out searches that have nothing to do with SQL Server or programming. Here are the results...
SQL SERVER BEGIN MONTH
xp_cmdshell
sql server" AND aggregate AND uniqueidentifier
northwind db
free sql code help
backup log files
Date formatting in SQL Server
union in sql
sql commands
show all tables sql statment
JDBC WITH SQL SERVER
determining server requirements
update row number sql
SQL functions in MS SQL Server
invoke xp_cmdshell from java
Login failed for user 'sa' Reason: not associated with a trusted SQL server connection
storage space" " text datatype"
what is an SQL server?
SQL server characteristic
application OLAP SQL 2000 with ASP
To see the top searches for October click here
SQL SERVER BEGIN MONTH
xp_cmdshell
sql server" AND aggregate AND uniqueidentifier
northwind db
free sql code help
backup log files
Date formatting in SQL Server
union in sql
sql commands
show all tables sql statment
JDBC WITH SQL SERVER
determining server requirements
update row number sql
SQL functions in MS SQL Server
invoke xp_cmdshell from java
Login failed for user 'sa' Reason: not associated with a trusted SQL server connection
storage space" " text datatype"
what is an SQL server?
SQL server characteristic
application OLAP SQL 2000 with ASP
To see the top searches for October click here
Friday, December 02, 2005
SQL Server 2005 Sample Book Chapters
Karen's SQL Blog has a blog post with a list of SQL Server 2005 sample chapters. Since I did not want to duplicate or copy the work that she has put into it I have decided to provide a link to that specific blog post here. Take a look around on her blog there are always links to interesting articles/downloads on her blog and it's updated frequently.
Thursday, December 01, 2005
Mapping SQL Server 2000 System Views To SQL Server 2005
SQL Server 2000 System Table SQL Server 2005 System View
syscolumns.....................sys.columns
syscomments....................sys.sql_modules
sysconstraints.................sys.check_constraints
...............................sys.default_constraints
...............................sys.key_constraints
...............................sys.foreign_keys
sysdepends.....................sys.sql_dependencies
sysfilegroups..................sys.filegroups
sysfiles.......................sys.files
sysforeignkeys.................sys.foreignkeys
sysfulltextcatalogs............sys.fulltextcatalogs
sysindexes.....................sys.indexes
sysindexkeys...................sys.index_columns
sysmembers.....................sys.databases_role_members
sysobjects.....................sys.objects
syspermissions.................sys.database_permissions
...............................sys.server_permissions
sysprotects....................sys.database_permissions
...............................sys.server_permissions
sysreferences..................sys.foreign_keys
systypes.......................sys.types
sysusers.......................sys.database_principals
Subscribe to:
Posts (Atom)